Contents
- What is Jlama?
- Use Cases for Jlama
- Why Use Jlama for LLM Inference in Java?
- Key Advantages of Jlama
- Real-World Impact of Jlama
- How to Set Up Jlama in Your Java Project?
- Troubleshooting Common Issues
- Best Practices for Optimizing LLM Inference with Jlama
- Real-World Applications of Jlama
- Comparison: Jlama vs. Other LLM Inference Engines
- Unique Advantages of Jlama
- Troubleshooting and Common Pitfalls
- The Future of LLM Inference in Java with Jlama
- Advancements in Jlama
- Key Takeaways from This Guide
- Ready to Get Started?
- Other Resources
What if you could effortlessly integrate cutting-edge AI into your Java applications to transform their functionality and efficiency?
Large language models (LLMs) are reshaping industries, enabling innovations like smart chatbots, automated content creation, and advanced text analysis. Despite their potential, Java developers often encounter significant barriers when adopting these technologies—issues like compatibility, resource management, and scalability often slow progress.
Enter Jlama, a Java-based LLM inference engine that breaks down these barriers. Designed for efficiency and ease of integration, Jlama empowers developers to unlock the capabilities of LLMs without needing deep AI expertise or extensive infrastructure modifications. By bridging the gap between advanced AI and Java’s robust ecosystem, Jlama ensures that LLM-powered solutions are within reach for all Java applications.
In this guide, we’ll explore how Jlama simplifies LLM inference, examine its key features and use cases, and provide actionable steps to set it up in your projects. Whether you’re enhancing customer experiences with AI or automating repetitive tasks, Jlama can help you achieve your goals with less effort and more impact.
Why should you keep reading?
By the end of this guide, you’ll understand how to:
- Leverage Jlama to boost your Java applications with LLMs.
- Set up and optimize Jlama for high performance and scalability.
- Explore practical applications and see how Jlama outshines competitors.
Let’s dive in!
What is Jlama?
Simplifying LLM Integration for Java Developers – Jlama is a cutting-edge inference engine designed to make large language models (LLMs) accessible to Java developers. Tailored for efficiency and ease of use, Jlama bridges the gap between advanced machine learning capabilities and the robust, scalable Java ecosystem.
By offering a lightweight, production-ready solution, Jlama enables seamless integration of LLMs into Java applications, allowing developers to focus on creating innovative solutions without being bogged down by complex AI implementation challenges.
Key Features of Jlama
- Lightweight Design – Jlama is optimized for minimal resource consumption, ensuring it can run efficiently on both on-premises systems and cloud environments. Its compact architecture makes it suitable for applications with tight performance constraints.
- High Performance – Engineered for speed, Jlama delivers low-latency inference, making it ideal for real-time applications like conversational AI, personalized recommendations, and data analysis.
- Java Native – Built specifically for Java, Jlama integrates effortlessly with popular Java frameworks such as Spring Boot, Jakarta EE, and Micronaut. This compatibility reduces the learning curve and simplifies deployment.
- Flexible Deployment – Whether you’re deploying in a microservices architecture, containerized environment, or serverless framework, Jlama adapts to your preferred setup with ease.
- Support for Pre-Trained Models – Jlama works seamlessly with a variety of pre-trained LLMs, including GPT-based models and domain-specific alternatives, enabling rapid deployment without extensive training requirements.
Use Cases for Jlama
- E-commerce – Leverage Jlama to build personalized shopping experiences through AI-driven product recommendations and responsive chatbots.
- Healthcare – Use LLMs to analyze patient records, automate report generation, and assist in diagnostics.
- Finance – Automate fraud detection, generate market analysis reports, and optimize customer support interactions.
- Customer Support – Create AI-driven chatbots capable of understanding and responding to user queries in natural language, improving resolution rates and user satisfaction.
- Content Creation – Automate the generation of summaries, reports, or creative content to save time and resources.
By addressing key industry challenges and enabling practical use cases, Jlama empowers Java developers to take full advantage of the latest advancements in AI.
Why Use Jlama for LLM Inference in Java?
Elevating Java Applications with the Power of LLMs – For Java developers, integrating large language models (LLMs) can be both an opportunity and a challenge. While LLMs bring capabilities like natural language understanding and automated text generation, the complexity of adapting these technologies to Java environments often limits their adoption. Jlama eliminates these barriers by providing a robust, optimized inference engine that caters specifically to Java applications.
Key Advantages of Jlama
1. Optimized Performance for Java Environments
Jlama is engineered to leverage the strengths of the Java runtime, ensuring seamless execution with minimal latency. It’s designed to handle the demanding computational requirements of LLMs while maintaining a smooth user experience.
Example: A chatbot built with Jlama can process user inputs and deliver accurate responses in milliseconds, ensuring real-time interaction.
2. Seamless Compatibility
Unlike generic AI tools, Jlama is tailored for Java ecosystems. It integrates effortlessly with popular Java frameworks, reducing development time and complexity.
Supported frameworks: Spring Boot, Jakarta EE, and Micronaut.
3. Scalability for Enterprise Applications
Jlama’s architecture is built for scale, making it ideal for high-traffic scenarios. Whether you’re deploying an e-commerce recommendation system or a healthcare diagnostic tool, Jlama ensures stable performance even under heavy load.
4. Cost Efficiency
By optimizing memory usage and computation, Jlama reduces infrastructure costs. It ensures efficient use of resources, whether running locally or in the cloud, making LLM adoption financially viable.
Comparison: Jlama consumes significantly less memory compared to Python-based frameworks, reducing cloud expenses for applications processing high volumes of data.
5. Ease of Use
With intuitive APIs and comprehensive documentation, Jlama minimizes the learning curve for developers. Its focus on usability allows teams to quickly prototype, test, and deploy applications powered by LLMs.
Real-World Impact of Jlama
Here’s how Jlama’s features translate into tangible benefits:
- Increased Productivity: Developers can implement powerful AI features without needing extensive training in machine learning.
- Enhanced User Experience: Faster, more accurate applications improve customer satisfaction and engagement.
- Business Scalability: Jlama’s cost-effective scalability supports growth without adding prohibitive expenses.
How to Set Up Jlama in Your Java Project?
A Step-by-Step Guide to Getting Started – Integrating Jlama into your Java project is a straightforward process, whether you’re working on a small prototype or a large-scale production application. This step-by-step guide will walk you through the setup process, ensuring you can start leveraging the power of LLMs with minimal effort.
Step 1: Add Jlama to Your Project Dependencies
Jlama is available via popular Java build tools like Maven and Gradle. Add the following dependency to your project’s configuration file:
For Maven:
<dependency>
<groupId>com.jlama</groupId>
<artifactId>jlama-engine</artifactId>
<version>1.0.0</version>
</dependency>
For Gradle:
implementation ‘com.jlama:jlama-engine:1.0.0’
Step 2: Initialize Jlama in Your Application
Start by importing the Jlama engine into your project and initializing it:
import com.jlama.LLMEngine;
public class JlamaApp {
public static void main(String[] args) {
LLMEngine engine = new LLMEngine();
System.out.println(“Jlama Engine Initialized!”);
}
}
Step 3: Load a Pre-Trained Model
Jlama supports a variety of pre-trained models. Download a compatible model and load it into the engine:
engine.loadModel(“path/to/pretrained-model”);
System.out.println(“Model loaded successfully!”);
Step 4: Process Input Data
Use the engine to process input text and generate outputs. For instance, creating a simple chatbot:
String input = “What is the capital of France?”;
String response = engine.infer(input);
System.out.println(“Response: ” + response);
Step 5: Configure Advanced Settings (Optional)
For production environments, optimize Jlama by configuring advanced settings like batch size, threading, and memory usage:
engine.setBatchSize(8);
engine.enableMultiThreading(true);
System.out.println(“Optimized for production!”);
Troubleshooting Common Issues
- Model Not Found: Ensure the file path to your model is correct.
- Memory Errors: Adjust batch size or use hardware acceleration like GPUs for larger models.
- Dependency Conflicts: Verify that Jlama’s dependencies are compatible with your project.
Best Practices for Optimizing LLM Inference with Jlama
After setting up Jlama, the next step is to ensure it performs optimally for your specific use case. By following these best practices, you can reduce latency, improve resource utilization, and scale effectively.
1. Hardware Optimization
Efficient hardware utilization is crucial for high-performance LLM inference. Jlama supports both CPUs and GPUs, but choosing the right configuration can significantly enhance speed.
- Leverage GPUs or TPUs: Use GPU acceleration for faster inference, especially with larger models.
- Cloud Integration: For scalable deployments, integrate Jlama with cloud services offering GPU/TPU support (e.g., AWS, GCP).
- Configuration Example:
engine.enableGPU(true);
System.out.println(“GPU acceleration enabled.”);
2. Memory Management
Large language models can consume substantial memory. Efficient resource allocation ensures stable performance:
- Adjust Batch Size: Optimize the batch size to balance memory usage and throughput.
- Enable Caching: Use Jlama’s built-in caching mechanisms to reuse frequent computations.
- Example:
engine.setBatchSize(16);
engine.enableCaching(true);
3. Parallel Processing
Jlama’s multithreading capabilities allow you to handle multiple requests simultaneously, improving throughput:
Enable Multithreading:
engine.enableMultiThreading(true);
Optimize Threads: Experiment with thread pool sizes to find the best configuration for your hardware.
4. Model Fine-Tuning
For domain-specific tasks, fine-tune pre-trained models to improve their relevance and accuracy:
- Fine-Tuning Steps:
- Prepare a dataset relevant to your domain.
- Use Jlama’s APIs to train on the dataset.
- Save and load the fine-tuned model.
- Example:
engine.fineTuneModel(“path/to/dataset”, “path/to/save/model”);
System.out.println(“Model fine-tuned successfully!”);
5. Monitor and Log Performance
Track key performance metrics such as latency, memory usage, and error rates to identify bottlenecks:
Enable Logging:
engine.enableLogging(true);
Analyze Logs: Use logs to identify and resolve performance issues.
6. Load Balancing for High-Traffic Applications
For applications with heavy traffic, implement load balancing to distribute requests across multiple instances of Jlama:
- Use a load balancer like NGINX or AWS Elastic Load Balancer.
- Deploy multiple instances of your application in a containerized environment (e.g., Kubernetes).
Real-World Applications of Jlama
Jlama is more than an inference engine—it’s a gateway to innovative solutions across industries. By integrating LLMs into Java applications, businesses can automate processes, enhance user experiences, and derive valuable insights from unstructured data. Let’s explore some practical applications of Jlama and how it addresses real-world challenges.
1. E-commerce: Personalization at Scale
- Challenge: Customers expect personalized shopping experiences, but manual customization is time-consuming.
- Solution: Jlama powers recommendation engines that analyze customer preferences and browsing behavior to suggest relevant products in real time.
- Example:
String input = “Customer recently purchased: laptop, headphones”;
String recommendation = engine.infer(input);
System.out.println(“Recommended products: ” + recommendation);
2. Healthcare: Enhanced Patient Care
- Challenge: Managing vast amounts of patient data and providing accurate diagnostics is complex.
- Solution: Use Jlama to process and summarize patient records or assist in preliminary diagnoses by analyzing symptoms and medical history.
- Example:
String input = “Patient symptoms: fever, cough, fatigue”;
String diagnosis = engine.infer(input);
System.out.println(“Preliminary diagnosis: ” + diagnosis);
3. Finance: Automating Reports and Analysis
- Challenge: Generating detailed financial reports and identifying patterns in market trends is resource-intensive.
- Solution: Jlama automates the generation of financial summaries and provides actionable insights by processing unstructured financial data.
- Example:
String input = “Analyze market trends for Q4 2023”;
String report = engine.infer(input);
System.out.println(“Market Analysis: ” + report);
4. Customer Support: AI-Powered Chatbots
- Challenge: Scaling customer support while maintaining quality interactions.
- Solution: Deploy Jlama to create chatbots that understand natural language queries and provide accurate, timely responses.
- Example:
String input = “How can I reset my password?”;
String response = engine.infer(input);
System.out.println(“Chatbot response: ” + response);
5. Content Creation: Automating Text Generation
- Challenge: Creating high-quality content consistently requires significant time and resources.
- Solution: Use Jlama to automate the generation of product descriptions, summaries, and blog posts, enabling faster content production.
- Example:
String input = “Write a product description for a smart home speaker”;
String output = engine.infer(input);
System.out.println(“Generated content: ” + output);
6. Education: Personalized Learning Tools
- Challenge: Adapting learning materials to individual student needs.
- Solution: Jlama enables the creation of intelligent tutoring systems that generate custom quizzes, explanations, and summaries based on student input.
- Example: Generating Custom Quizzes
String input = “Create a quiz on basic algebra with 5 questions”;
String quiz = engine.infer(input);
System.out.println(“Generated Quiz: ” + quiz);
Comparison: Jlama vs. Other LLM Inference Engines
How Does Jlama Stand Out in the World of LLM Inference? The market for LLM inference engines offers a range of tools, each with its strengths and weaknesses. However, Jlama is uniquely positioned for Java developers, combining high performance, seamless integration, and cost efficiency. Let’s compare Jlama with popular alternatives to see how it stacks up.
Key Comparison Factors
1. Performance
Jlama is optimized for Java environments, delivering low-latency inference even for large models. Its architecture takes advantage of Java’s efficient runtime to reduce bottlenecks.
Benchmark Comparison:
Jlama Advantage:
- Lower latency than Python-based solutions.
- More memory-efficient than TensorFlow for large-scale applications.
2. Compatibility
Unlike general-purpose inference engines, Jlama is built for Java, ensuring seamless integration with frameworks like Spring Boot and Jakarta EE.
Jlama Advantage:
- Fully compatible with Java frameworks, reducing setup time and simplifying deployments.
3. Ease of Use
Jlama offers an intuitive API and well-documented setup, making it easy for developers to integrate LLMs into projects without extensive AI expertise.
Developer Feedback:
- Jlama: “Simple to set up and use, with clear documentation tailored for Java developers.”
- Hugging Face: “Powerful, but Python-centric and less intuitive for Java users.”
- TensorFlow Serving: “Requires deep ML knowledge and infrastructure expertise.”
4. Scalability and Cost Efficiency
Jlama’s lightweight design allows it to scale efficiently while minimizing resource consumption.
- Handles high traffic with minimal performance degradation.
- Reduces infrastructure costs by using memory and compute resources effectively.
Unique Advantages of Jlama
- Java First: The only LLM inference engine built specifically for Java developers.
- Production-Ready: Optimized for real-world applications, from small prototypes to enterprise-grade systems.
- Cost-Effective Scalability: Ensures businesses can grow without overspending on infrastructure.
- Ease of Deployment: Integrates with existing Java environments with minimal disruption.
Key Scenarios Where Jlama Excels
- Java-Only Ecosystems: Ideal for organizations heavily invested in Java technologies.
- Resource-Constrained Applications: Efficient memory and CPU utilization make it suitable for on-premises setups.
- Real-Time Systems: Low-latency performance ensures smooth user interactions in applications like chatbots and recommendation engines.
Troubleshooting and Common Pitfalls
Even with its ease of use and optimized design, developers may encounter challenges when implementing Jlama. This section addresses common pitfalls and provides actionable solutions to ensure a seamless experience.
1. Model Not Loading Properly
- Symptom: The application throws an error when attempting to load a model, or the model fails to initialize.
- Common Causes:
- Incorrect file path to the model.
- Using an unsupported model format.
- Insufficient permissions to access the model file.
- Solution:
- Verify the file path and ensure it is correct.
- Check that the model format is compatible with Jlama (e.g., .bin or .onnx).
- Update file permissions using a command like chmod if necessary.
- Example code for loading a model:
engine.loadModel(“path/to/pretrained-model”);
System.out.println(“Model loaded successfully!”);
2. High Latency During Inference
- Symptom: Inference requests take longer than expected, leading to delays in real-time applications.
- Common Causes:
- Insufficient hardware resources (e.g., CPU, RAM, GPU).
- High batch sizes causing memory overhead.
- Network latency if using a remote model server.
- Solution:
- Enable GPU acceleration for faster processing:
engine.enableGPU(true);
- Reduce batch size to optimize memory usage:
engine.setBatchSize(8);
- Use a local model deployment for latency-critical applications
3. Out-of-Memory Errors
- Symptom: The application crashes or throws memory allocation errors during inference.
- Common Causes:
- Loading a model too large for available system memory.
- Running inference with overly large inputs.
- Solution:
- Use a smaller or optimized version of the model if available.
- Break input data into smaller chunks for processing:
String input = “Large text input split into smaller parts.”;
String response = engine.infer(input);
- Allocate more memory to your Java Virtual Machine (JVM) by adjusting the heap size:
java -Xmx4G -jar your-application.jar
4. Dependency Conflicts
- Symptom: Dependency-related errors or runtime exceptions during application execution.
- Common Causes:
- Conflicts between Jlama dependencies and other project libraries.
- Solution:
- Check for dependency conflicts using tools like Maven Dependency Analyzer.
- Use dependency exclusions to resolve conflicts:
<dependency>
<groupId>com.jlama</groupId>
<artifactId>jlama-engine</artifactId>
<version>1.0.0</version>
<exclusions>
<exclusion>
<groupId>conflicting.library.group</groupId>
<artifactId>conflicting-library</artifactId>
</exclusion>
</exclusions>
</dependency>
5. Unexpected Output from the Model
- Symptom: The model generates irrelevant or incorrect responses during inference.
- Common Causes:
- Using an incorrect or generic pre-trained model for a domain-specific task.
- Input data format or structure not aligned with the model’s training data.
- Solution:
- Fine-tune the model with domain-specific data:
engine.fineTuneModel(“path/to/domain-dataset”, “path/to/save/model”);
- Preprocess input data to ensure compatibility with the model’s training format.
6. General Debugging Tips
- Enable Logging: Turn on detailed logging to identify issues:
engine.enableLogging(true);
- Consult Documentation: Use the Jlama documentation for troubleshooting common issues.
- Community Support: Join Jlama forums or GitHub discussions for peer support.
The Future of LLM Inference in Java with Jlama
As large language models continue to evolve, their applications are expanding into new domains and industries. Jlama is at the forefront of this transformation, enabling Java developers to harness the full potential of LLMs in an efficient and scalable way. Let’s explore what the future holds for LLM inference in Java and how Jlama is shaping this landscape.
Advancements in Jlama
1. Enhanced Model Support
- Jlama is set to expand its compatibility with state-of-the-art models, including domain-specific LLMs for healthcare, finance, and more.
- Planned support for larger, more complex models with optimized resource usage.
2. Improved Performance
- Upcoming releases will focus on reducing latency even further, leveraging innovations in Java’s runtime optimization and hardware acceleration.
- Incremental updates for better multithreading and batch processing capabilities.
3. Built-In Fine-Tuning Tools
- Future updates will include streamlined tools for fine-tuning models directly within Jlama, eliminating the need for external frameworks.
- Enhanced support for integrating fine-tuned models into production environments.
4. Integration with Emerging Technologies
- Native support for federated learning and edge deployments, allowing for privacy-preserving and distributed AI applications.
- Seamless integration with cloud AI services for hybrid infrastructure setups.
Trends in LLM Inference and Java Development
- Democratization of AI – As tools like Jlama become more accessible, we’ll see broader adoption of LLMs in industries where Java dominates, such as finance, enterprise software, and IoT.
- AI-Powered Java Frameworks – Future Java frameworks are likely to integrate LLM capabilities as a core feature, with Jlama leading the way as the inference engine of choice.
- Sustainable AI Practices – With increasing focus on sustainability, Jlama’s resource-efficient design will play a key role in reducing the environmental impact of large-scale AI deployments.
Opportunities for Developers
- Community Contributions – Developers can contribute to Jlama’s growth by participating in its open-source initiatives, building plugins, or improving its core features.
- Training Programs and Certifications – As Jlama gains traction, specialized training programs and certifications will help developers master LLM inference in Java.
- New Use Cases – With continuous enhancements, Jlama will unlock innovative applications, from real-time data analysis in financial trading to AI-driven automation in industrial IoT.
Embracing the Future with Jlama
The synergy between Java and LLMs is just beginning, and Jlama is leading this exciting frontier. By simplifying complex AI processes and delivering exceptional performance, Jlama is empowering developers to redefine the possibilities of Java applications.
Whether you’re looking to enhance existing software or explore new horizons, Jlama ensures you’re ready to meet the demands of the next generation of AI-powered solutions.
Conclusion
Integrating large language models into Java applications no longer needs to be a complex or resource-intensive endeavor. With Jlama, developers can leverage the power of LLMs to enhance functionality, automate tasks, and deliver exceptional user experiences—all while staying within the familiar Java ecosystem.
Key Takeaways from This Guide
- What Jlama Offers: A lightweight, high-performance LLM inference engine tailored specifically for Java, enabling seamless integration and scalability.
- Why Jlama Stands Out: Optimized for Java frameworks, cost-efficient, and equipped with an intuitive API, making it the perfect choice for Java developers.
- Real-World Applications: From e-commerce personalization and AI-powered chatbots to education and finance, Jlama transforms ideas into impactful solutions.
- Best Practices: By leveraging GPUs, optimizing memory management, and fine-tuning models, you can maximize Jlama’s performance in demanding scenarios.
- Future Outlook: With its commitment to innovation and developer-centric design, Jlama is poised to lead the next wave of AI in Java applications.
Ready to Get Started?
Now that you’re equipped with the knowledge to implement Jlama, it’s time to take the next step. Whether you’re building a prototype or scaling an enterprise application, Jlama offers the tools and performance you need to succeed.
- Download Jlama and integrate it into your project today.
- Explore the documentation for detailed guidance and best practices.
- Join the Jlama community to connect with other developers and share your experiences.
Other Resources
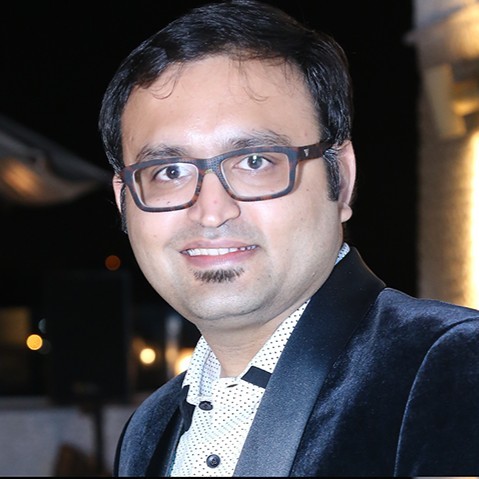
Director of Engineering | CTO | Software Developer
As a passionate technologist and seasoned software developer, I specialize in creating innovative solutions at the intersection of AI and software engineering. With a keen interest in leveraging the latest technologies to solve real-world problems, my work spans developing advanced AI models to designing scalable software architectures. Beyond coding, I contribute to tech blogs, sharing insights on AI trends, software best practices, and the future of technology. My mission is to empower organizations and individuals through technology, driving forward the boundaries of what’s possible